리액트를 학습하고 로그인, 로그아웃, 회원가입 페이지를 다뤄본 적이 없어서 경험을 해볼 겸 학습을 시작하였다.
먼저 리액트에선 프론트를 담당하고 db와 연결 및 데이터 검증을 해줄 서버 구성이 필요했다.
기존에 해보던 Java로 해도 괜찮지만 NodeJS를 적용해보면 간편하고 쉬울 것 같아서 시작하게 되었다.(새PC에 자바가 없어서 세팅하기가 귀찮다는 그런 이유도 있다...)
🚀 목표
- 리액트로 로그인 페이지 구현
- 로그아웃 처리
- 회원가입 기능
- 노드JS에서 REST API 처리
- DB연결 및 쿼리 작성
- 세션 처리
💡 생각해 볼 것
로그인 처리 후 인증 방식에 크게 Session
과 JWT
가 있다.
아직 JWT
를 경험하지 못해서 학습할 겸 적용해봐도 좋지만 노드JS도 다뤄본적이 없는데 너무 헤멜것 같아서 먼저 이번 목표를 수행하고 다음 과제로 수행 해 볼 예정이다. 고로 클래식한 Session
처리로 한다.
🔧 설치할 모듈
express
: nodeJS에서 서버를 구성하기 위한 라이브러리nodemon
: node기반에서 변경사항이 발생하면 자동으로 재시작해주는 라이브러리cocurrently
: 명령어를 동시에 실행시켜준다.(node, react동시 실행용)react & typescript
: 리액트(ts.ver) 설치http-proxy-middleware
: 프록시 설정을 위해 필요한 라이브러리(react -> node로 fetch때 사용예정)mysql2
: mysql접속을 위해 설치(설정 문제 때문에 2로 설치하였다.)
⚙️ 환경 설정
서버 구성하기(NodeJS )
먼저 서버로 작업할 디렉토리를 하나 파고 아래의 명령을 수행한다.
$ npm init -y
$ npm install express nodemon concurrently mysql2
이것만으로 가볍게 웹서버를 구성할 수 있다.
서버로 사용할 index
페이지를 생성한다.
📄index.js
const express = require('express');
const app = express();
const port = process.env.PORT || 3001;
app.listen(port, function () {
console.log(`server running on ${port}`);
});
nodemon
설정을 위해 package.json을 수정한다.
📄package.json
"scripts": {
"start": "nodemon index.js"
}
프론트 생성(리액트 ts.ver)
$ npx create-react-app client --template typescript
리액트(client)에서 프록시 설정을 위해 아래 명령어로 라이브러리를 설치한다.
$ cd client
$ npm install http-proxy-middleware
설치가 끝나면 setupProxy
파일을 생성하고 아래와 같이 입력한다.
📄/client/src/setupProxy.js
const { createProxyMiddleware } = require("http-proxy-middleware");
module.exports = function (app) {
app.use(
createProxyMiddleware("/login/**", {
target: "http://localhost:3001",
changeOrigin: true,
})
);
};
개발 실행 환경 구성하기
package.json을 손봐야 vscode하나를 켜놓고 서버와 리액트를 동시에 실행하여 편하게 개발할 수 있다.
scripts부분을 아래와 같이 추가한다. (리액트쪽 package.json이 아님!!)
📄package.json
"scripts": {
"start": "nodemon index.js",
"dev": "concurrently \"npm run dev:server\" \"npm run dev:client\"",
"dev:server": "npm start",
"dev:client": "cd client && npm start"
}
앞으로 개발시 npm run dev
를 통해 개발 테스트를 할 수 있다.
🔥 임시 작성 테스트 결과
리액트 설정
📄/client/src/login/login.tsx
import { useEffect, useState } from "react";
export default function Login() {
const [userId, setUserId] = useState("");
const [userPw, setUserPw] = useState("");
const handleInput = (e: React.ChangeEvent<HTMLInputElement>) => {
if (e.target.name === "userId") {
setUserId(e.target.value);
} else if (e.target.name === "userPw") {
setUserPw(e.target.value);
}
};
const goLogin = () => {
console.log("click!");
};
useEffect(() => {
fetch("/login/test")
.then((res) => res.json())
.then((data) => console.log(data));
}, []);
return (
<div>
<h2>Login Test</h2>
<div>
<label htmlFor="">아이디</label>
<input
type="text"
name="userId"
value={userId}
onChange={handleInput}
/>
</div>
<div>
<label htmlFor="">비밀번호</label>
<input
type="password"
name="userPw"
value={userPw}
onChange={handleInput}
/>
</div>
<div>
<button type="button" onClick={goLogin}>
로그인
</button>
</div>
</div>
);
}
📄src/App.tsx
import React from "react";
import Login from "./components/login/login";
function App() {
return (
<div>
<Login />
</div>
);
}
export default App;
서버 설정
📄index.js
const express = require("express");
const app = express();
const port = process.env.PORT || 3001;
app.listen(port, function () {
console.log(`server running on ${port}`);
});
app.use("/login/test", function (req, res) {
res.json({ test: "success" });
});
테스트 캡처
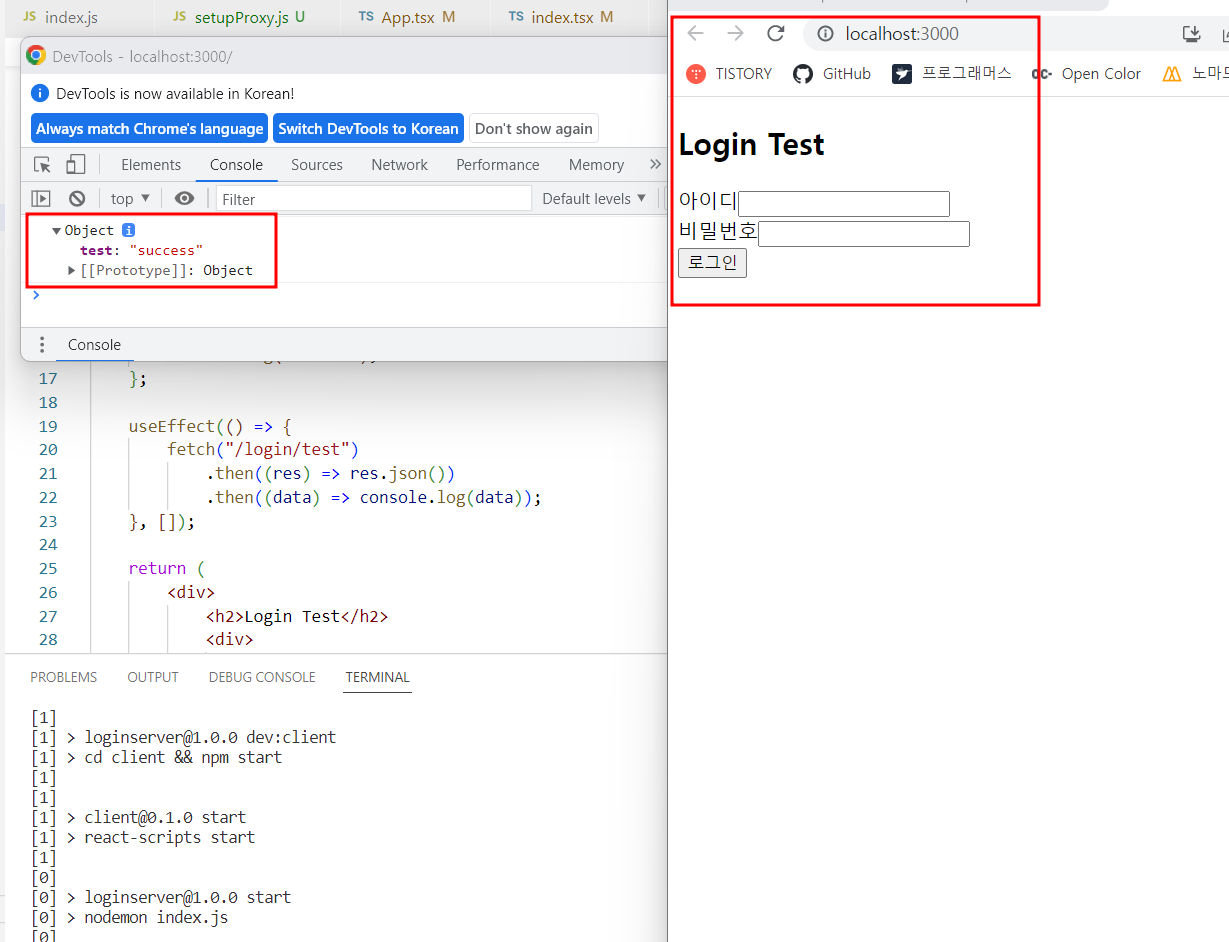
login/test로 부터 응답을 받아서 클라이언트쪽에서 콘솔 로그에 결과값이 잘 찍히고 있다.
'WEB > React' 카테고리의 다른 글
React - Node JS와 로그인 연동 해보기03(뷰 추가) (0) | 2023.05.25 |
---|---|
React - Node JS와 로그인 연동 해보기02(서버 추가 설정) (0) | 2023.05.25 |
React - firebase 개행 처리하기 (0) | 2023.03.27 |
React - 구글 폰트 적용하기(styled-component Global에러) (0) | 2023.03.15 |
React - Style component font 적용방법 알아보기(typescript.ver) (0) | 2023.03.15 |